Service History
API
Understand a vehicle’s service history
Our Service History endpoint allows developers to access data about a vehicle's past service appointments, including cost and service notes.
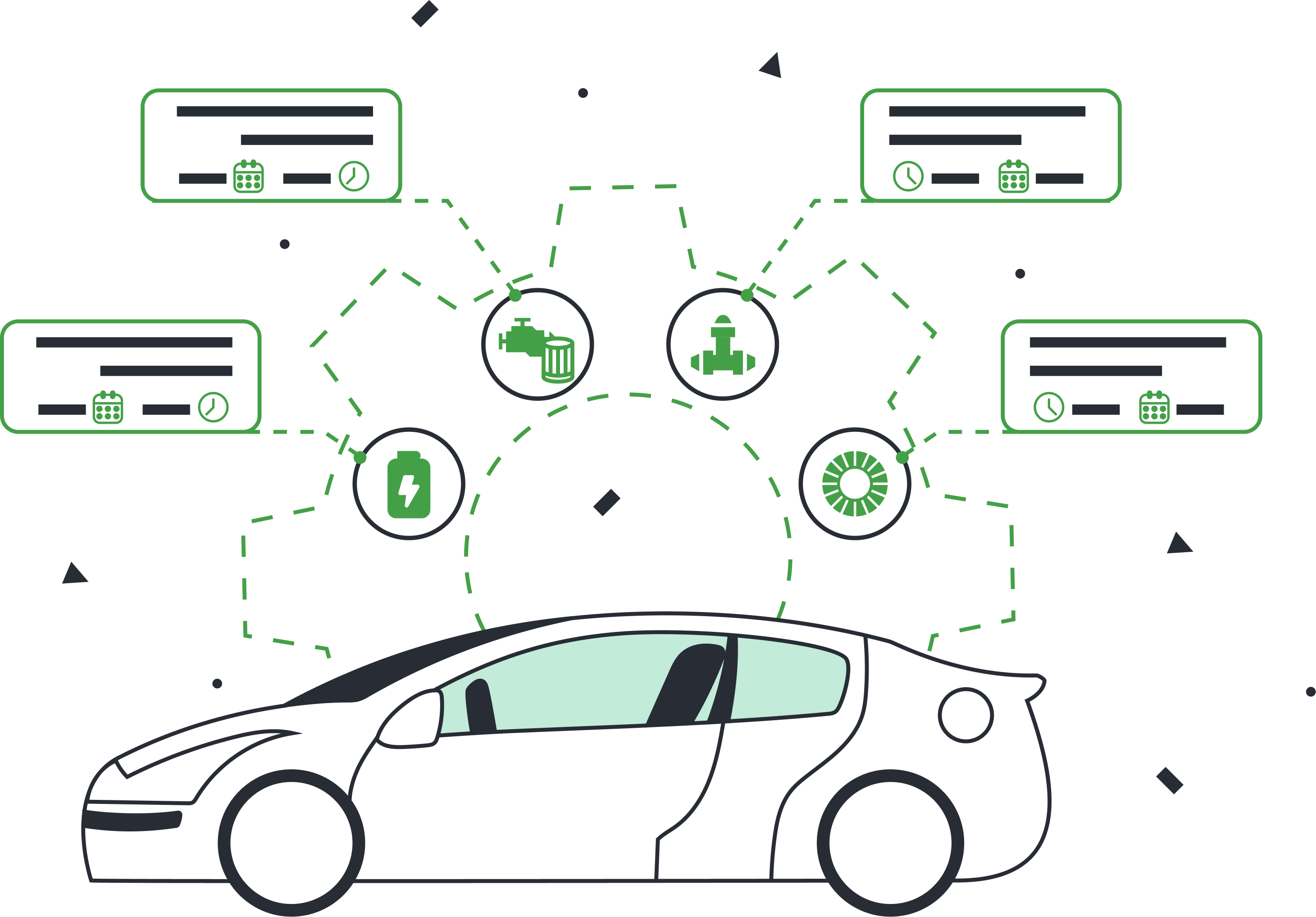
How businesses use service history
Independent repair shops
Understand prior maintenance for better future service scheduling to see when a vehicle was last serviced and for what.
Auto insurance
Set accurate rates based on a vehicle’s service history and how well the vehicle has been maintained.
Car dealerships
Access detailed service reports, to see a vehicle's previous upkeep or issues. Improved valuation, accurate history data enable data-driven pricing and competitive lending rates.
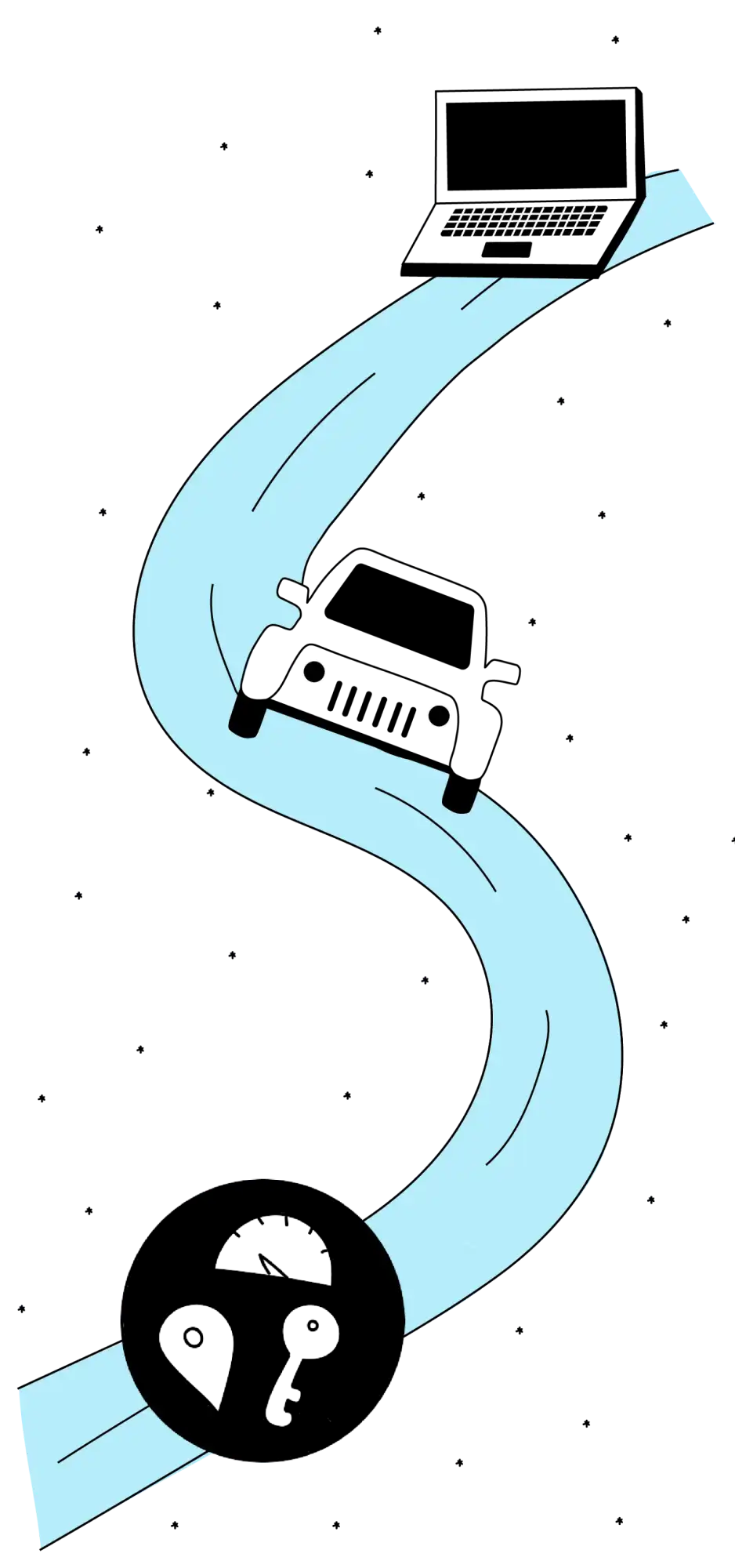
Service History
Retrieve service records tracked by the vehicle’s dealer or manually added by the vehicle owner.
Product features
Compatible with 39 car brands
Friendly user consent flow
Works on 2015 and newer vehicles
Trusted & secure
Access to live car data
SDKs for Go, Java, Node.js, Python, and Ruby
Related industries
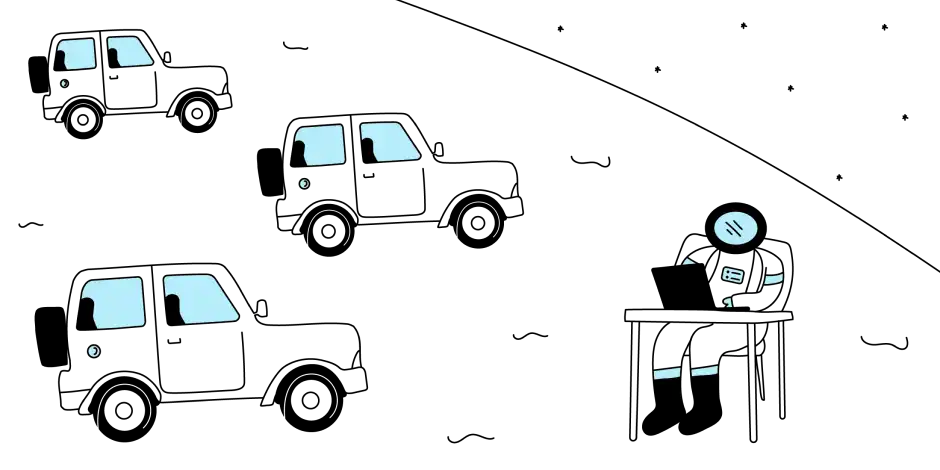
Fleet management
Offer automatic engine oil life checks as part of your fleet management software and predictive maintenance products.
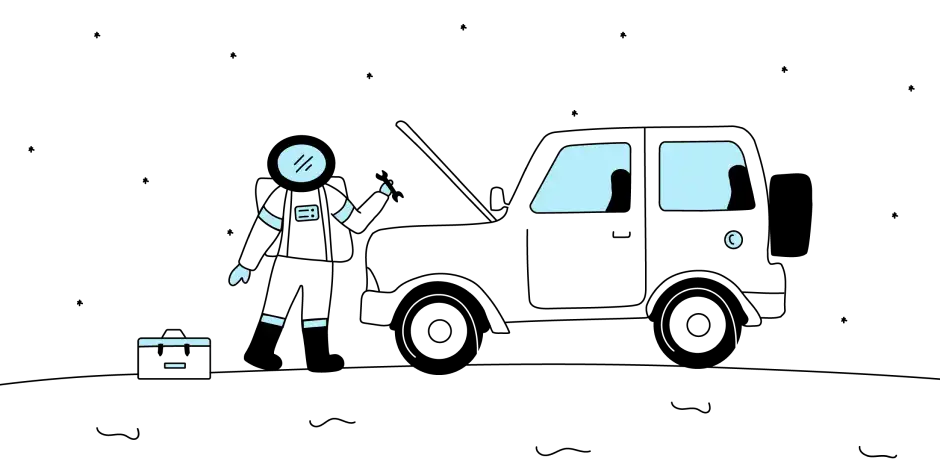
On-demand services
Invite customers to schedule a maintenance visit when their vehicle’s engine oil reaches the end of its recommended life span.
What we’re building
Latency and frequency of data availability may vary between makes and models.