EV battery
API
Klicken, zustimmen, SoC abrufen.
Lesen Sie den Batteriestand und die Batteriekapazität eines Elektrofahrzeugs mit einfachen API-Anfragen ab.

Ladezustand der Batterie
Rufen Sie den Ladezustand (SoC) und die verbleibende Reichweite eines batterieelektrischen Fahrzeugs (BEV) oder eines Plug-in-Hybridfahrzeugs (PHEV) ab.
Kapazität der Batterie
Informieren Sie sich über die Kapazität der Batterie eines Elektrofahrzeugs.
Eigenschaften des Produkts
kompatibel mit 39 Automarken
Benutzerfreundlicher Zustimmungsfluss
Funktioniert mit Fahrzeugen von 2015 und neueren
Vertrauenswürdig und sicher
Zugriff auf Live-Fahrzeugdaten
SDKs für Go, Java, Node.js, Python und Ruby
Verwandte Branchen
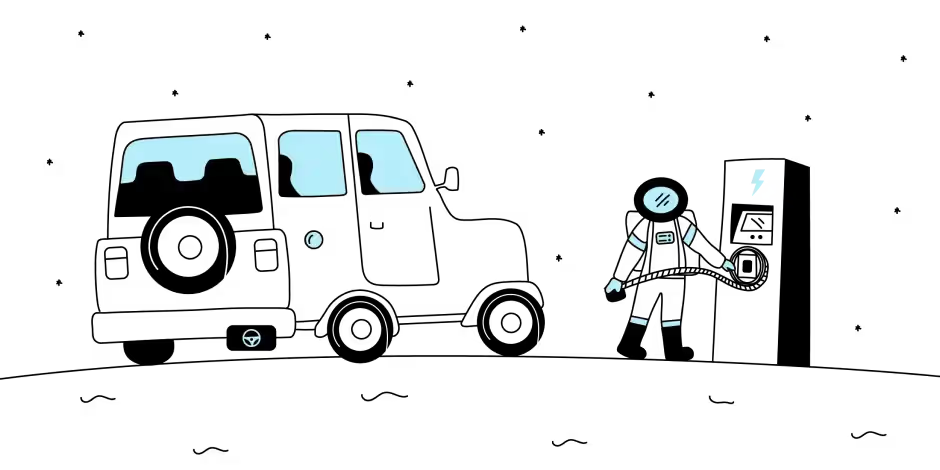
Ladenetzwerke für Elektrofahrzeuge
Geben Sie in Ihrer App geschätzte Ladezeiten, automatische Ladepläne und die Reiseplanung für Elektrofahrzeuge an.

Energie- und Versorgungsunternehmen
Steuern Sie das Laden der Elektrofahrzeuge Ihrer Kunden in Privathaushalten, um die Stromnetzlast optimal auszugleichen.
Was wir bauen
Latenz und Häufigkeit der Datenverfügbarkeit können je nach Hersteller und Modell variieren.