Vehicle attributes
API
Greifen Sie auf wichtige Fahrzeugdaten zu
Informieren Sie sich über Marke, Modell und Baujahr des Autos Ihres Kunden.

Marke, Modell und Jahr
Ruft Marke, Modell und Modelljahr eines Fahrzeugs ab.
Eigenschaften des Produkts
kompatibel mit 39 Automarken
Benutzerfreundlicher Zustimmungsfluss
Funktioniert mit Fahrzeugen von 2015 und neueren
Vertrauenswürdig und sicher
Zugriff auf Live-Fahrzeugdaten
SDKs für Go, Java, Node.js, Python und Ruby
Verwandte Branchen
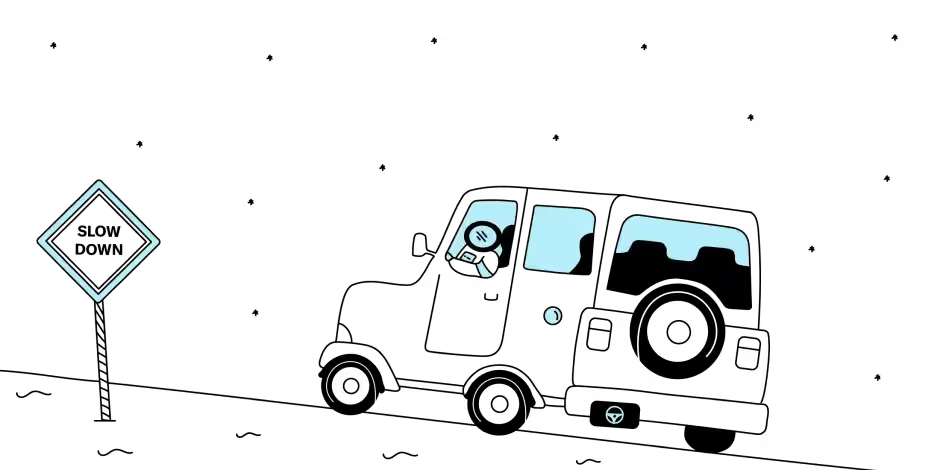
Autoversicherung
Bestätigen Sie die Marke, das Modell und das Jahr des Fahrzeugs Ihres Kunden, um falsch angegebene Versicherungsinformationen zu erkennen.
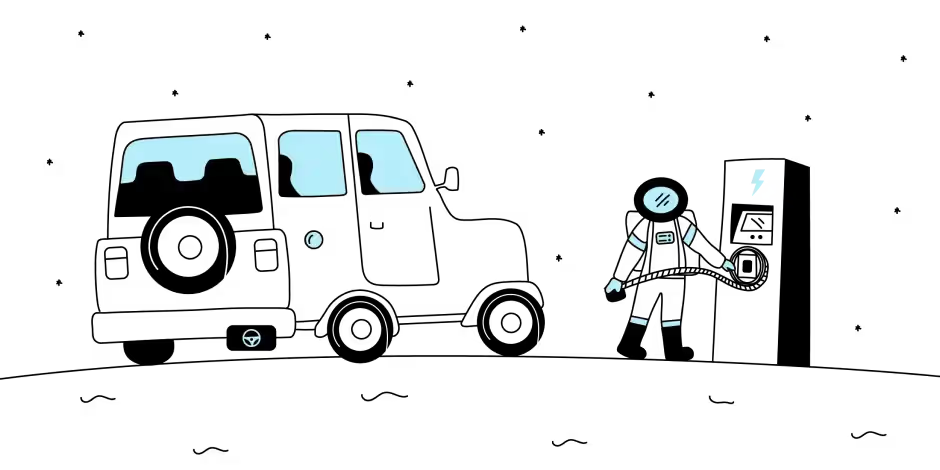
Ladenetzwerke für Elektrofahrzeuge
Erfahren Sie mehr über das Fahrzeug, das Ihr Kunde fährt, und bieten Sie ihm personalisierte Ladedienste für Elektrofahrzeuge an.
Was wir bauen
Latenz und Häufigkeit der Datenverfügbarkeit können je nach Hersteller und Modell variieren.